Sandbox Testing
This guide describes how to test in-app purchases in your app and provides useful tips.
Android
Testing purchases in Android is the same as real purchases. However, you will need to add your Google account to application license testing in order to make purchases for free. For more information about the testing purchases, please read the official documentation.
Test a Fresh Install
To test a fresh install on Android you will need to:
- Delete your user in Apphud
- Delete the app
Android SDK doesn't save the User ID / Device ID to the device keychain. This means that deleting the app and user in Apphud will generate a new User ID / Device ID pair. When launching the app after re-install, you will get a fresh user without purchases.
However, if restore purchases are called, Apphud will merge an existing user with a new user. As a result, the User ID will change to the original one and the user will have two devices, with the old and new Device IDs. The original purchase history will be restored.
Clear Purchase History
Google Play doesn't fully clear purchase history. The best way is to change your Google account on the device, but if this is not an option, you can refund / revoke transactions in Google Play console > Order management
Find your transaction that your recently purchased and click Refund. Don't forget to remove entitlement, so it won't appear in the recent purchases list.
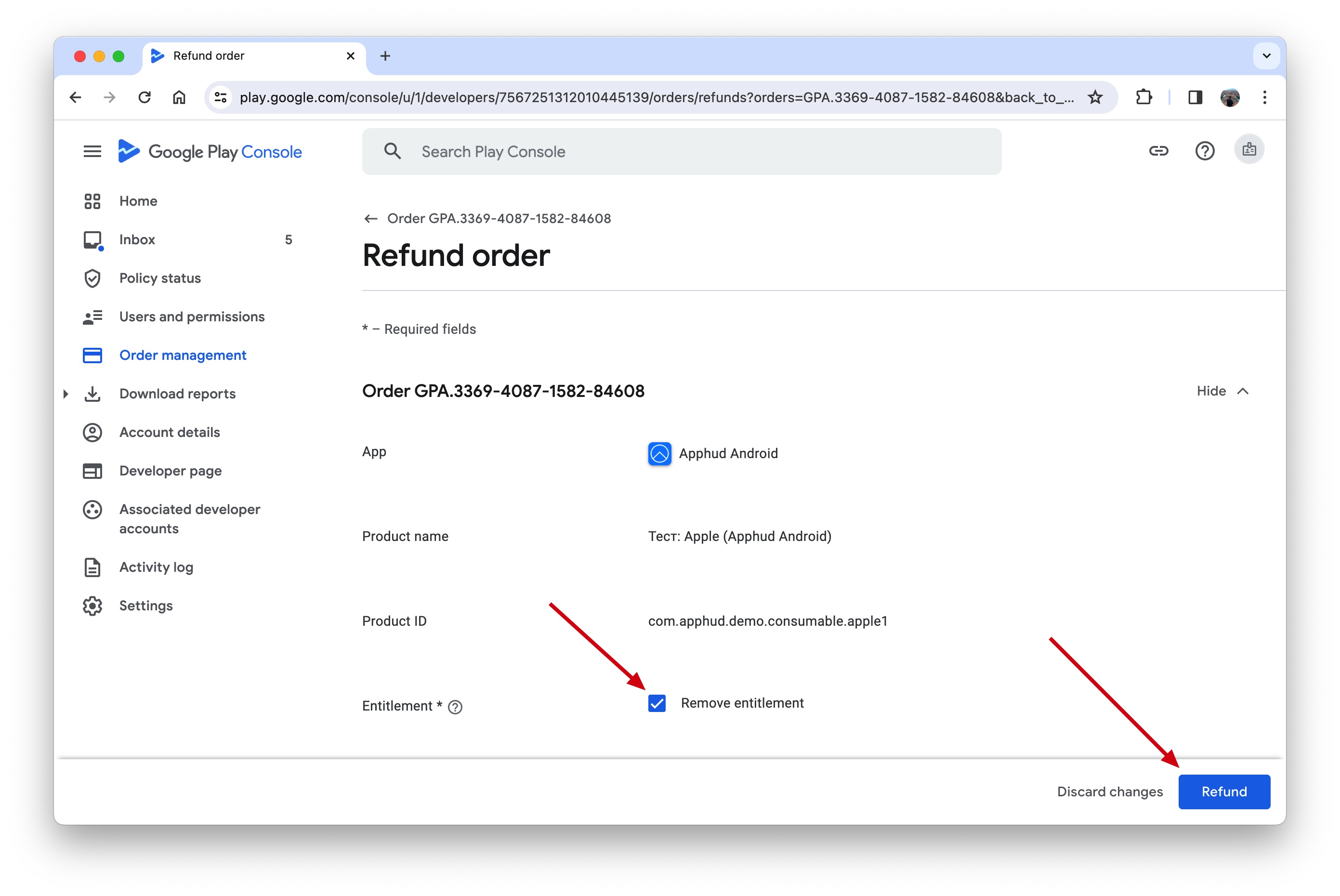
iOS
Test a Fresh Install
To ensure a clean testing environment for a fresh install scenario on iOS using Apphud, it's essential to remove any previous associations with sandbox purchases. Follow these steps to achieve a fresh install experience:
- Delete Your User in Apphud: Start by removing your user profile within Apphud. This step is crucial to disassociate any previous in-app purchases tied to your account, ensuring that subsequent tests do not inherit active purchases.
- Delete the App: Uninstall the app from your device. This action clears locally stored data and settings related to the app, further ensuring that the upcoming installation is treated as a first-time download and install.
- Clear Purchase History for Sandbox Builds: Specifically for tests conducted with sandbox builds, navigate to your Sandbox Account Settings and execute a clear-out of the purchase history. This action removes any record of past transactions conducted under your sandbox account, preventing them from influencing new tests.
Testing Environments
There are three ways to test purchases in iOS:
- Local StoreKit Testing (Xcode build using StoreKit Configuratio file). Can make purchases in iOS simulator. Doesn't have purchase history. Re-installing the app is enough.
- Sandbox Testing. Requires creating Sandbox Account in App Store Connect. The main advantage is that you can clear purchases history in iOS Settings > App Store > Sandbox Account.
- TestFlight Testing . TestFlight builds use real Apple ID. In-App Purchases made using TestFlight build cannot be cleared.
You can read more about differences and limitations between Sandbox and StoreKit Testing in this documentation.
TestFlight Testing
TestFlight allows you to distribute your app to up to 10,000 testers using just their email address. It's ideal for testing with a larger, more diverse group of users, including those who are not part of the development team.
Important
It is not possible to clear purchase history in TestFlight builds.
Local StoreKit Testing
Apphud supports receipts made using StoreKit Configuration File. No need to update SDK and no additional code is required. With StoreKit Configuration File enabled in your scheme, you can make purchases in iOS Simulator (or real device) as usual by calling Apphud.purchase(product){...}
method. You can read more about StoreKit Configuration File set up in our blog post.
Note, that receipt generated by Local StoreKit is limited, and doesn't contain many fields that are available when verifying receipts through Apple. For example, trial period
field is missing, as well as pending_renewal_info
, which is a special sub-json containing important fields about the state of subscription, like auto-renew, in billing retry, etc.
Limitations
- Unable to detect
trial
status of the subscription. That means after purchasing subscription with trial period, you will getApphudSubscripton
model withregular
status in the response. But don't worry, that is only local receipt issue, everything will work fine in production! ApphudSubscripton
models generated from local receipts will always haveisInRetryBilling
=false
andisAutorenewEnabled
=true
, unless expired.- Simplified renewals logic. Local receipt is just a snapshot and we are unable to detect renewals of such receipts, until a fresh local receipt is sent to our backend. Which means that subscription will move to
expired
state after the first transaction is expired. When launching the app again in simulator, a new receipt will be automatically sent to Apphud, and subscriptions data will be updated. No actions required on developer side. Again, everything will work just fine in production. - Apphud doesn't create transactions and events of local receipts. In Apphud User page, subscription will only have status and expiration date.
- Testing promotional offers using Xcode generated Subscription Offers key will be supported soon.
- Testing eligibilities is not supported.
- Testing Apphud Rules is not supported.
Note
We don't validate local receipts with the StoreKit certificate at the moment (you can test without them, everything would work fine). The certificates required for testing automation scenarios. Will be added later.
Sandbox Testing
Ad Hoc Distribution
You can distribute apps that use Sandbox accounts via AdHoc distribution. This requires creating custom provisioning profile and selecting particular test devices. Learn more.
Use the Apple sandbox environment to test your implementation of in-app purchases that use the StoreKit framework on devices with real product information from App Store Connect. Your development-signed app uses the sandbox environment when you sign in to the App Store using a Sandbox Apple ID.
You build and run your app from Xcode. However, you can also distribute development-signed apps via Ad Hoc.
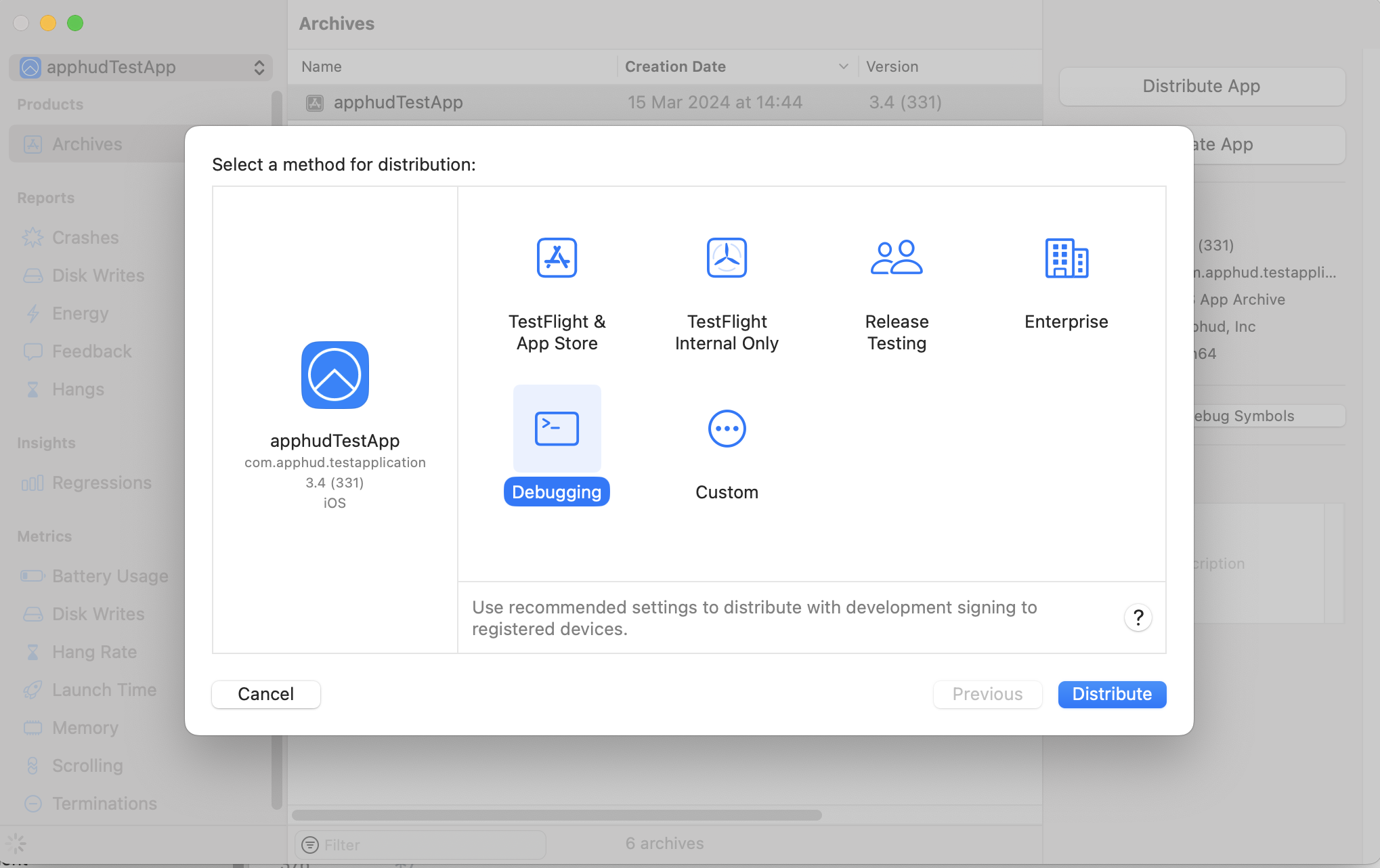
Select Debugging or Custom method for Sandbox distribution
Sandbox testing requires creating Sandbox User if testing in Xcode build. You can read more about differences and limitations between Sandbox and StoreKit Testing in this documentation.
Create Sandbox User
Creating a sandbox test user is required for making purchases in Xcode build. You can create as many sandbox users as you want in App Store Connect. In most cases one sandbox user is enough, but you may want to create a new sandbox user in order to clear purchases history.
Note
You can read more about testing and setting up auto-renewable subscriptions in our blog.
To test in app purchases you need to create sandbox user. Go to App Store Connect and open "Users and Access", then – "Sandbox Testers":
Manage Sandbox Account
You can manage sandbox account subscriptions and other parameters in your iOS Settings > App Store screen:
Clear Purchase History
In Sandbox Account Settings you can Clear Purchase History, manage accelerated renewal rate and manage subscriptions, i.e. cancel subscriptions, Reset introductory eligibiliy.
Cancel Sandbox Subscriptions
Here you can cancel subscriptions, upgrade or downgrade them, or even reset the introductory offer eligibility.
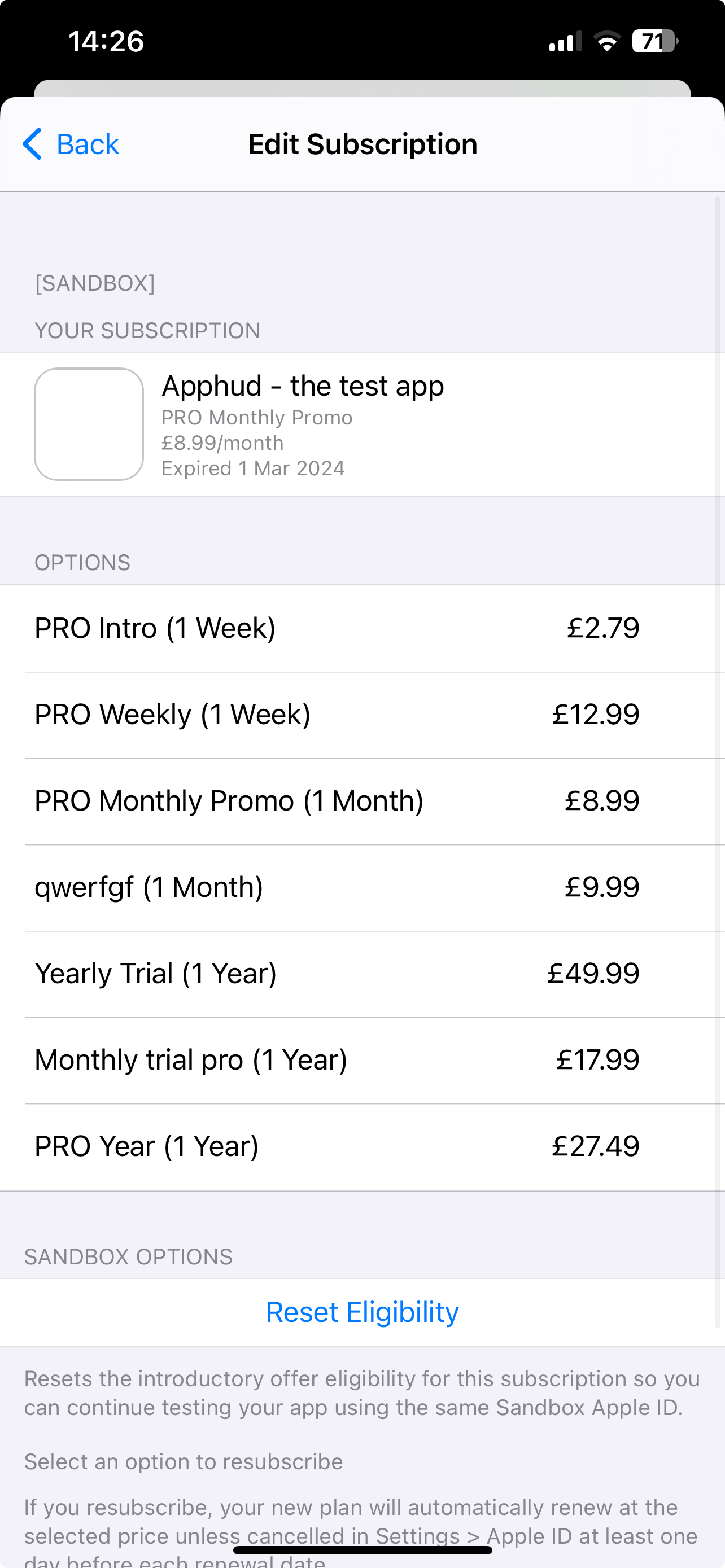
Sandbox Purchasing
Purchasing process is the same as real in-app purchases. The only difference is accelerated time and impossibility to cancel subscription manually. Subscriptions in sandbox renew 6 times per day maximum.
Note
You can read more about creating sandbox testers here.
Real duration | Duration in sandbox |
---|---|
1 week | 3 minutes |
1 month | 5 minutes |
2 months | 10 minutes |
3 months | 15 minutes |
6 months | 30 minutes |
1 year | 1 hour |
Which Events Can Be Tested?
You can test following all events in sandbox except REFUNDED events.
However, REFUNDED events can still be tested using Local StoreKit Testing.
Note
You can read more about events here.
Eligibilities
Important Note
Testing Eligibilities are not supported in Local StoreKit Testing mode. Use Sandbox testing instead.
Apphud checks whether the given user is eligible for purchasing introductory or promotional offers by searching for transactions in ANY of the current user's subscriptions. This means that if your Apphud user has several app store receipts from previous sandbox Apple IDs, you may get unexpected results.
Apphud User may have several subscriptions with the same product from a different Apple ID account if you were changing sandbox Apple ID without deleting Apphud User. In this case, Apphud will merge multiple subscriptions under the same user. That is why it is recommended to delete your Apphud User from Apphud Dashboard when testing the app from another Apple ID.
Testing Eligibility for Introductory Offer
To correctly use the checkEligibilityForIntroductoryOffer
method please do the following:
- Please make sure that your Apphud User doesn't contain multiple App Store receipts from previous test Apple IDs. If so, delete your user.
- Note that the "Reset Eligibility" option (Settings > App Store > Sandbox Account > Manage > Your App) is not supported. Apple doesn't delete old trial transactions from the receipt, so there is no technical way to correctly determine introductory eligibility after you pressed the "Reset Eligibility" button.
- Test on a clean Apple ID account. Create a fresh sandbox Apple ID account.
- When running the app for the first time after deleting (i.e. fresh install), keep in mind that you would need to submit App Store Receipt to Apphud either by calling
Apphud.migratePurchasesIfNeeded{}
or by tapping the restore purchases button in your App's UI. This is required only in Sandbox because apps running from Xcode/TestFlight initially don't have App Store Receipts and Apphud doesn't automatically refresh missing App Store receipts to avoid Apple ID password prompt. At production, however, everything will work okay, because App Store receipts always exist in apps downloaded from the App Store. - Now you can test your eligibility method: the method will return
true
because you didn't yet use the introductory offer. After you have used the introductory offer, the method will returnfalse
.
Testing Eligibility for Promotional Offer
To correctly use checkEligibilityForPromotionalOffer
method please do the following:
- Please make sure that your Apphud User doesn't contain multiple App Store receipts from previous test Apple IDs. If so, delete your user.
- When running the app for the first time after deleting (i.e. fresh install), keep in mind that you would need to submit App Store Receipt to Apphud either by calling
Apphud.migratePurchasesIfNeeded{}
or by tapping the restore purchases button in your App's UI. This is required only in Sandbox because apps running from Xcode/TestFlight initially don't have App Store Receipts and Apphud doesn't automatically refresh missing App Store receipts to avoid Apple ID password prompt. At production, however, everything will work okay, because App Store receipts always exist in apps downloaded from the App Store. - Now you can test your promo eligibility method: the method will return
true
if Apphud User has any subscription andfalse
if the user doesn't have any subscriptions.
Troubleshooting
Having issues with sandbox purchases? See our Troubleshooting guide.
Updated 8 months ago