Push Notifications
This guide will help you to properly configure Push notifications in Apphud.
iOS Only
This guide applies to iOS only as Push Notifications is a part of Rules feature which is only available on iOS
Integrating Push notifications in your iOS app will let you use Rules – a powerful feature that lets you increase your app revenue by automatically offering a discount to a user at the specified moment.
Generate Push Notifications Auth Key
Go to the Apple Developer Center, then go to "Keys" page, then create a new key by entering a name and choosing "Apple Push Notifications service (APNs)":
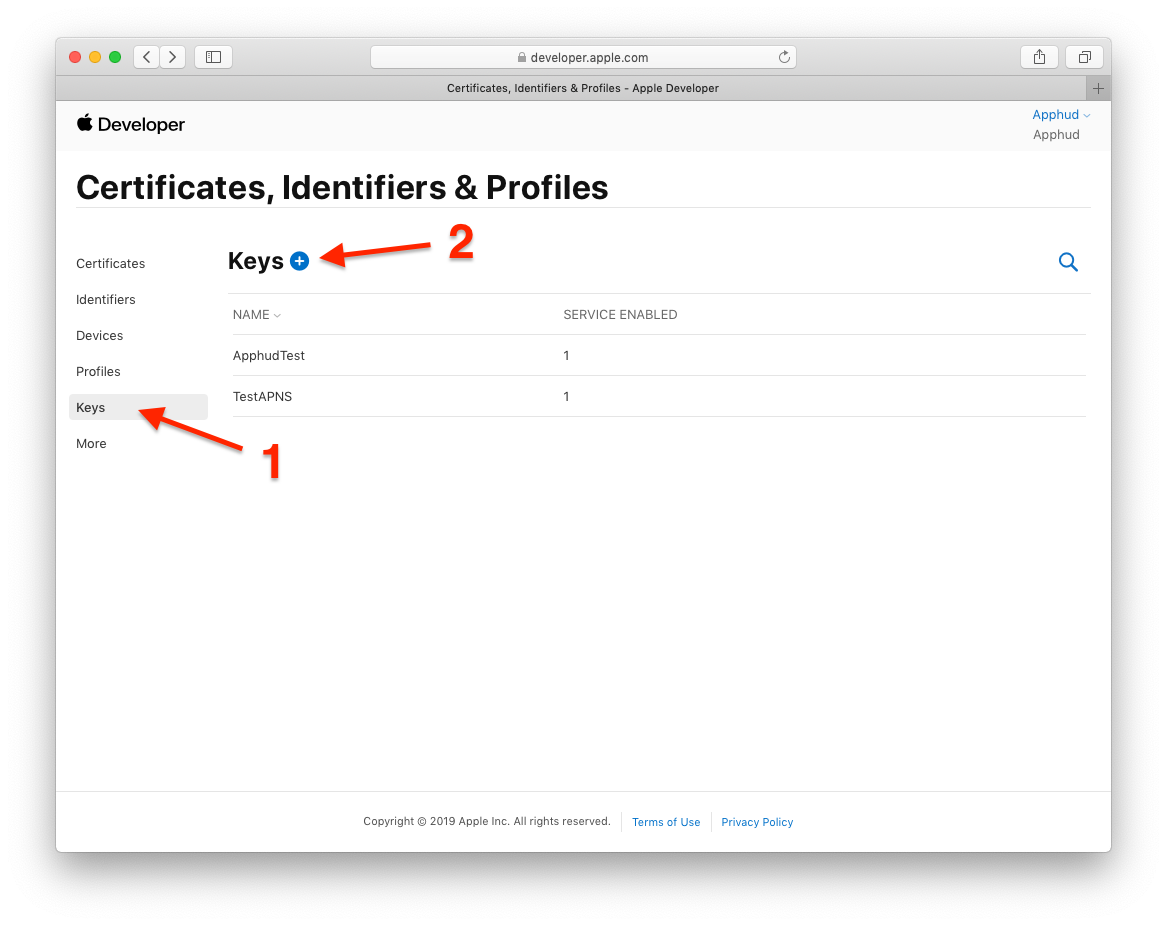
Once created, download the file and move it to a safe place. You will need to upload it to Apphud. Also, copy your team ID somewhere which can be found on your Developer Account Membership page. We will need it later.
Note
Auth Key file name has the following format:
AuthKey_[KEY_ID].p8
, whereKEY_ID
is your Key Identifier. By using auth key, Apphud will be able to send Push notifications to all of your apps within the same account and to both sandbox and production environments. That means you can use the same auth key file for your other apps.
Upload Auth Key to Apphud
Go to Apphud Dashboard and open "App settings", select "Push" tab. There you will see Upload button for APNs auth key file. Just upload your Key file there. Also, enter your Team ID and make sure key identifier is also entered_:_
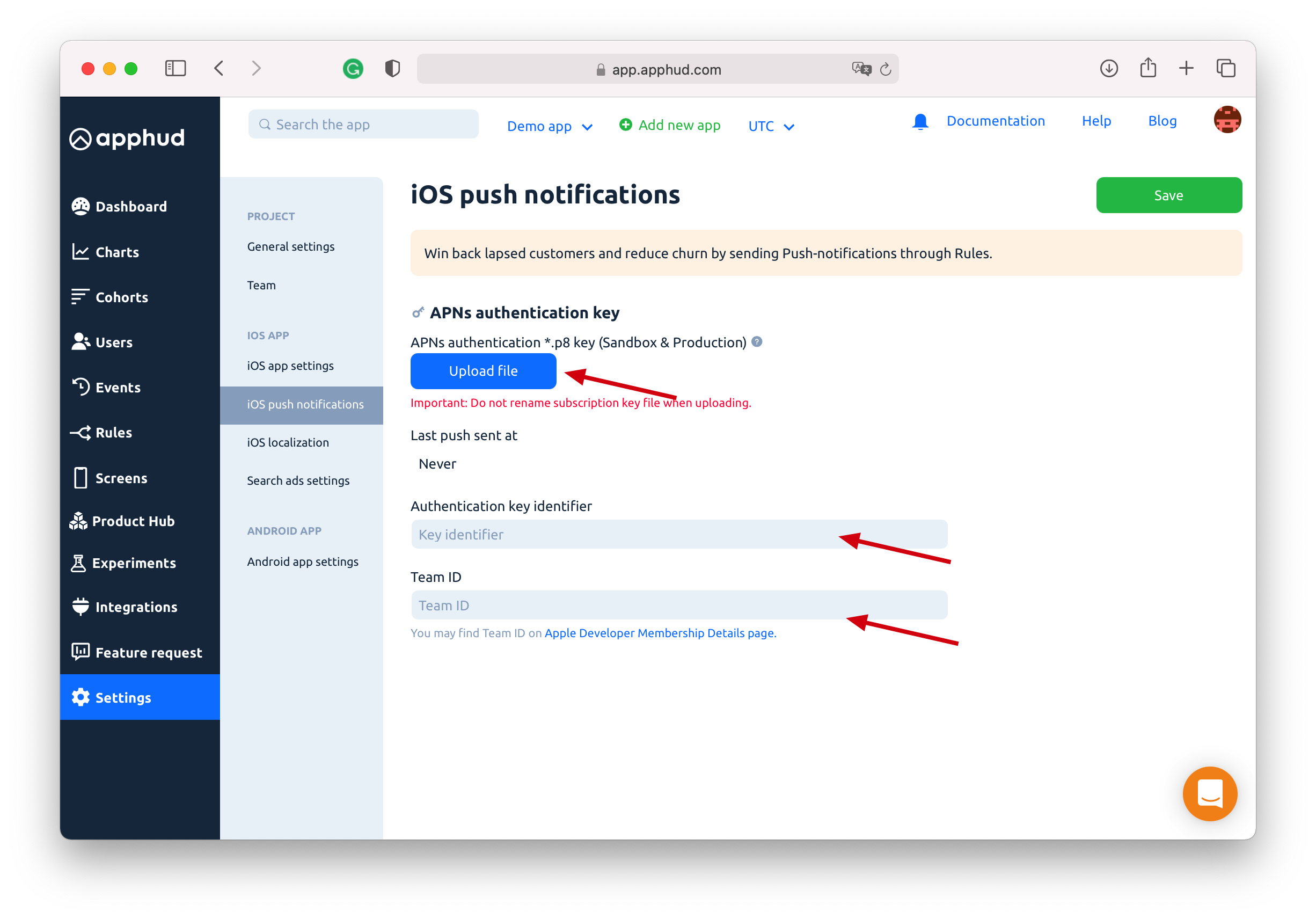
[Required] Set up Push Notifications in the iOS app
Please follow all the steps below.
Add Push Notifications Capability
Make sure that Push Notifications are turned on in "Capabilities" section of your app target:
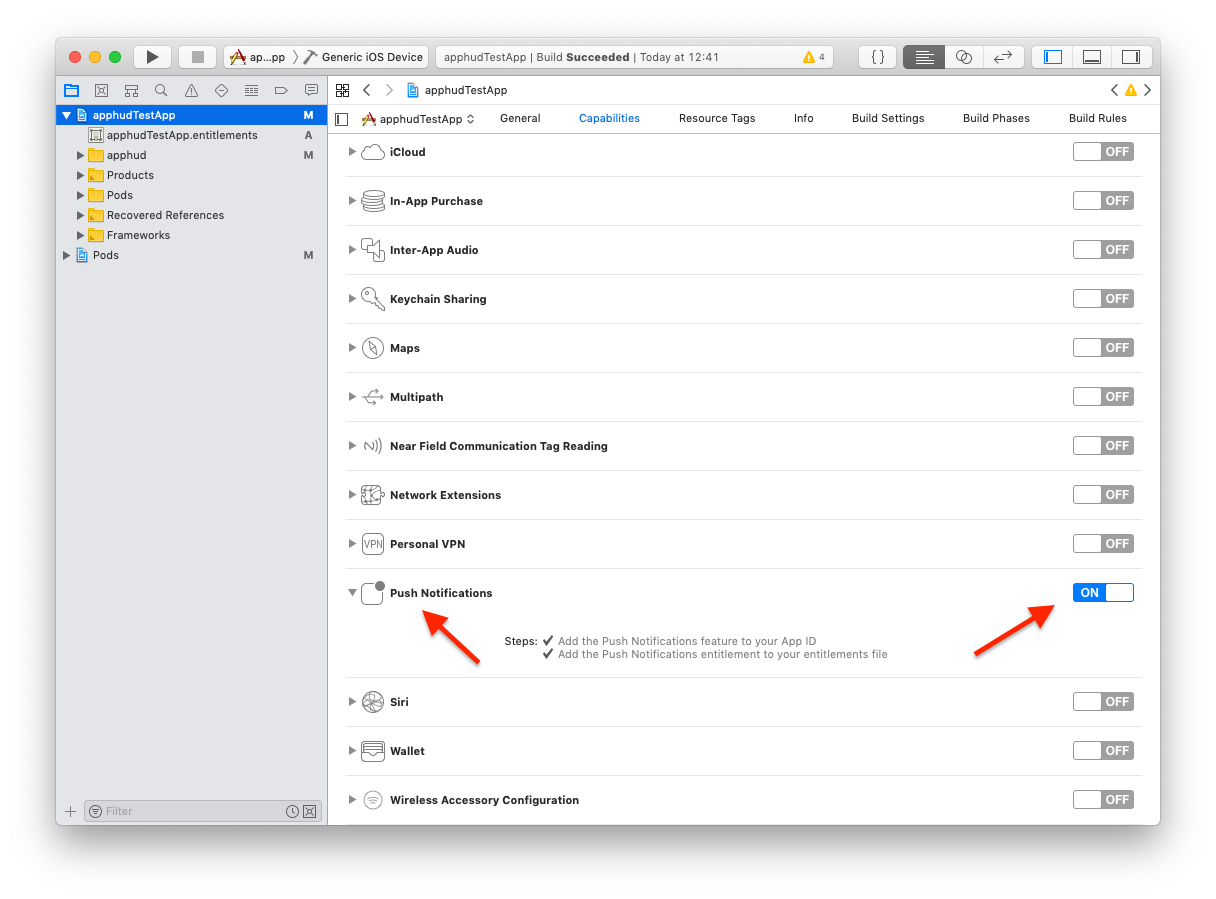
Register for Notifications
Let's write some code in your AppDelegate
. First, register for notifications:
import UserNotifications
func application(_ application: UIApplication, didFinishLaunchingWithOptions launchOptions: [UIApplication.LaunchOptionsKey: Any]?) -> Bool {
Apphud.start(apiKey: YOUR_API_KEY)
registerForNotifications()
//... the rest of your code
}
func registerForNotifications(){
UNUserNotificationCenter.current().delegate = self
UNUserNotificationCenter.current().requestAuthorization(options: [.alert, .badge, .sound]) { (granted, error) in
// handle if needed
}
UIApplication.shared.registerForRemoteNotifications()
}
#import <UserNotifications/UserNotifications.h>
- (BOOL)application:(UIApplication *)application didFinishLaunchingWithOptions:(NSDictionary *)launchOptions {
// Override point for customization after application launch.
[Apphud startWithApiKey:@"APPHUD_API_KEY" userID:nil];
[self registerForNotifications];
}
- (void)registerForNotifications{
UNUserNotificationCenter.currentNotificationCenter.delegate = self;
[UNUserNotificationCenter.currentNotificationCenter requestAuthorizationWithOptions:(UNAuthorizationOptionAlert | UNAuthorizationOptionBadge | UNAuthorizationOptionSound) completionHandler:^(BOOL granted, NSError * _Nullable error) {
// handle if needed
}];
[[UIApplication sharedApplication] registerForRemoteNotifications];
}
Pass Device Token to Apphud
Then you will need to submit the device token to Apphud:
func application(_ application: UIApplication, didRegisterForRemoteNotificationsWithDeviceToken deviceToken: Data) {
Apphud.submitPushNotificationsToken(token: deviceToken, callback: nil)
}
func application(_ application: UIApplication, didFailToRegisterForRemoteNotificationsWithError error: Error) {
// error occurred. Probably you have signing issues or push notifications capabilities are // turned off
}
- (void)application:(UIApplication *)application didRegisterForRemoteNotificationsWithDeviceToken:(NSData *)deviceToken{
[Apphud submitPushNotificationsTokenWithToken:deviceToken callback:nil];
}
- (void)application:(UIApplication *)application didFailToRegisterForRemoteNotificationsWithError:(NSError *)error{
// error occurred. Probably you have signing issues or push notifications capabilities are turned off
}
We should handle the incoming Push notification payload after. This is done with two methods: one handles payload when the app is in the background or not launched, and another – handles payload when the app is running.
Handle Incoming Push Payload
func userNotificationCenter(_ center: UNUserNotificationCenter, didReceive response: UNNotificationResponse, withCompletionHandler completionHandler: @escaping () -> Void) {
if Apphud.handlePushNotification(apsInfo: response.notification.request.content.userInfo) {
// Push Notification was handled by Apphud, probably do nothing
} else {
// Handle other types of push notifications
}
completionHandler()
}
func userNotificationCenter(_ center: UNUserNotificationCenter, willPresent notification: UNNotification, withCompletionHandler completionHandler: @escaping (UNNotificationPresentationOptions) -> Void) {
if Apphud.handlePushNotification(apsInfo: notification.request.content.userInfo) {
// Push Notification was handled by Apphud, probably do nothing
} else {
// Handle other types of push notifications
}
completionHandler([]) // return empty array to skip showing notification banner
}
- (void)userNotificationCenter:(UNUserNotificationCenter *)center didReceiveNotificationResponse:(UNNotificationResponse *)response withCompletionHandler:(void (^)(void))completionHandler {
[Apphud handlePushNotificationWithApsInfo:response.notification.request.content.userInfo];
}
- (void)userNotificationCenter:(UNUserNotificationCenter *)center willPresentNotification:(UNNotification *)notification withCompletionHandler:(void (^)(UNNotificationPresentationOptions))completionHandler {
[Apphud handlePushNotificationWithApsInfo:notification.request.content.userInfo];
}
That's it. Run the app and make sure that deviceToken
is successfully sent to Apphud.
Apphud SDK handles payload and returns true
if it was successfully handled in Apphud.handlePushNotification(apsInfo: userInfo)
method.
Now you are ready to receive Push notifications.
Push Notification Payload
Here is an example of a Push Notification Payload
aps = {
alert = {
body = TEST;
title = "Paywall!";
};
"mutable-content" = 1;
sound = default;
};
custom = {
a = {
};
};
"rule_id" = "5242761d-918d-42ae-a49b-f155b1402c2b";
"rule_name" = "Paywall Custom Rule";
"screen_id" = "0f8b832e-65d3-4777-a303-ba89750763c9";
"screen_name" = "your_screen_name";
}
"custom" subjson added to the payload, but it is not used. This is an internal subjson, which was added to handle conflicts with OneSignal SDK, if available.
Silent Push Notifications
Apphud supports sending silent push notifications delivered to the app in the background without alerting the user.
Silent push notifications:
- do not require user permission and are designed to be lightweight and non-intrusive;
- do not show alerts, sounds, or banners.
They’re ideal for background automation without interrupting your user.
These pushes are used for:
- Background data updates (e.g., refreshing subscription status)
- Running in-app logic quietly (like preparing personalized content)
- Updating app badge or local storage
- Supporting serverless automations via Rules
Please use this guide for more details.
Troubleshooting
Push notification doesn't arrive.
- Double-check that Auth Key is uploaded to App Settings in Apphud and that both Key ID and Team ID values are set.
- Make sure that Apphud receives device tokens from your iOS app, i.e. double check that
Apphud.submitPushNotificationsToken(token: deviceToken, callback: {_ in})
method is being called. - View your Apphud User page – it should contain push token value.
Push notification arrives but it's not being handled.
- Make sure that
Apphud.handlePushNotification(apsInfo: userInfo)
method is being called. - Make sure that
UNUserNotificationCenterDelegate
is correctly set. - If you are using OneSignal or custom
UINotificationServiceExtension
, debug in Xcode and make sure thatApphud.handlePushNotification(apsInfo: userInfo)
method is called.
Using both OneSignal and Apphud Push Notifications
Please see this guide.
Updated about 2 months ago