User Properties
This page describes how to set or update user properties
Set custom User Properties (or User Attributes) through SDK. These attributes will be displayed in Apphud User Page and can be segmented in charts and filters. For example, you can view revenue or MRR chart segmented by your own user attribute.
Features
- Set user property using system reserved or custom keys
- Increment user property with integer or floating value
- Set once, so it will not be overwritten.
Usage examples
Apphud.setUserProperty(key: .email, value: "[email protected]", setOnce: true)
Apphud.setUserProperty(key: .age, value: 31)
Apphud.setUserProperty(key: .init("custom_test_property_1"), value: true)
Apphud.setUserProperty(key: .gender, value: "male")
Apphud.setUserProperty(key: .init("custom_email"), value: "[email protected]", setOnce: true)
Apphud.incrementUserProperty(key: .age, by: 1)
Apphud.incrementUserProperty(key: .init("progress"), by: 0.5)
Apphud.setUserProperty(ApphudUserPropertyKey.Email, "[email protected]", true)
Apphud.setUserProperty(ApphudUserPropertyKey.CustomProperty("custom_increment_value_1"), 2.1f)
Apphud.setUserProperty(ApphudUserPropertyKey.Age, 29)
Apphud.incrementUserProperty(ApphudUserPropertyKey.CustomProperty("custom_increment_value_2"), 0.01f)
await AppHud.setUserProperty(key: ApphudUserPropertyKey.email, value:’[email protected]’ , setOnce:true);
await AppHud.setUserProperty(key: ApphudUserPropertyKey.age, value:31);
await AppHud.setUserProperty(key:ApphudUserPropertyKey.customProperty
(‘custom_test_property_1’), value:true);
await AppHud.setUserProperty(key:ApphudUserPropertyKey.gender, value:’male’);
await AppHud.setUserProperty(key:ApphudUserPropertyKey.customProperty
(‘custom_email’), value:’[email protected]’,setOnce:true);
await AppHud.incrementUserProperty( key: ApphudUserPropertyKey.age, by: 1,);
await AppHud.incrementUserProperty( key:ApphudUserPropertyKey.customProperty
(‘progress’), by: 0.5);
System Reserved User Properties
Key | Value |
---|---|
$email | String |
$phone | String |
$name | String |
$cohort | String |
$age | Int |
$gender | Must be one of male , female , other |
Limitations
- Value must be one of:
Int
,Float
,Double
,Bool
,String
,NSNumber
,NSString
,NSNull
,nil
ornull
. - Key must be 50 characters maximum.
- App may have maximum of 60 active attributes. If you overflow the limit, new properties will not be saved.
Manage User Properties
You can manage your current User Properties in the Settings > Custom User Properties tab. If you no longer using the property, you can disable it and it won't be count as active, and will be ignored in API calls.
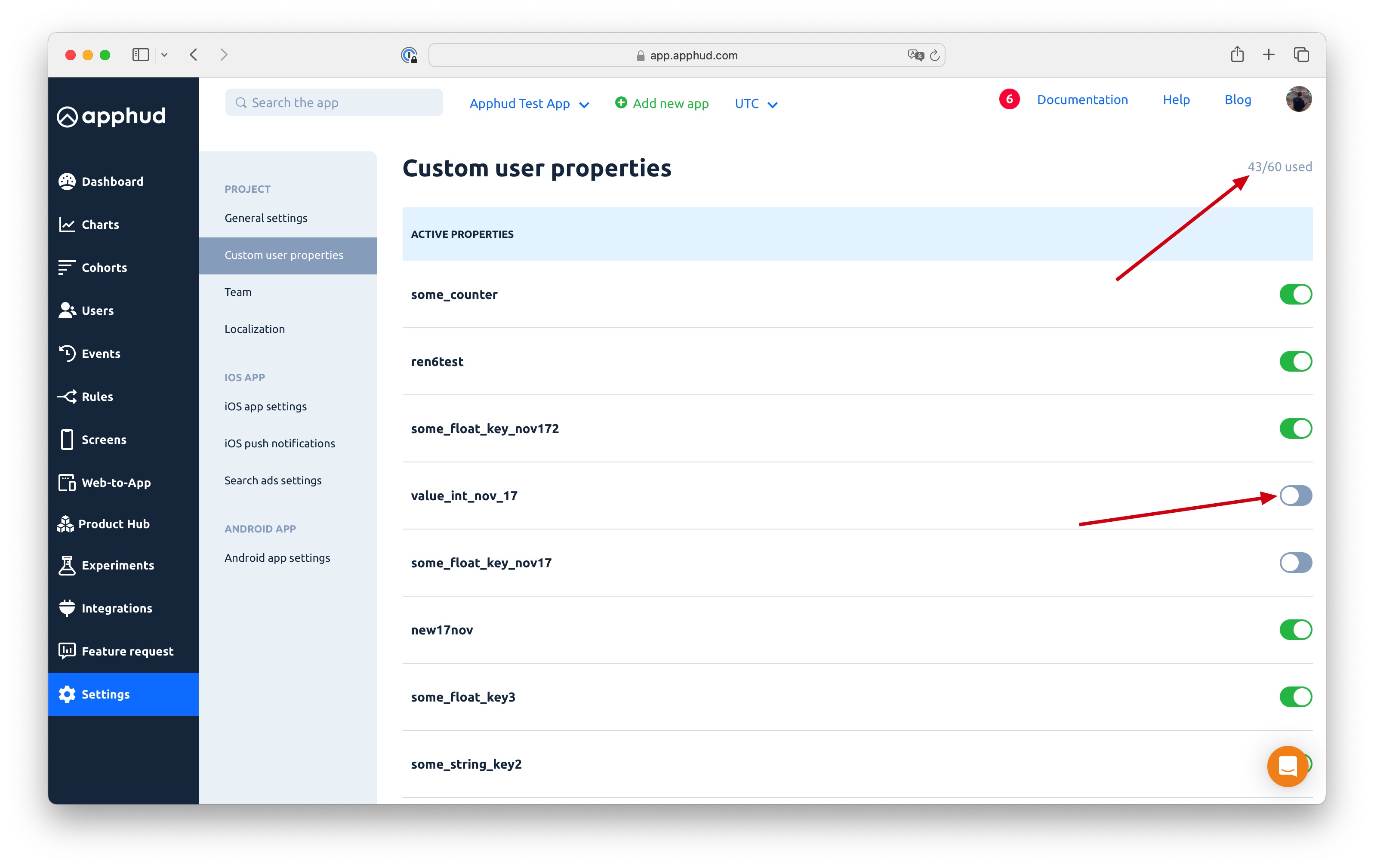
Custom User Properties Usage in Apphud
Analytics
Custom properties help expand the range of insights available in various charts and reports. By passing meaningful attributes, you can analyze users in ways that go beyond standard filters and segments.
For example, analyze revenue trends across different age groups or use answers from onboarding quizzes to see if initial preferences influence users' LTV. These insights can drive smarter decisions and improve overall app performance.
Note
Custom User Properties are available in Analytics Reports as Segmentations and Filters parameters on "Expert" and "Enterprise" plans.
Audience Creation Tips
Custom properties can also be used to define audiences, which can then be targeted in Placements, Experiments, and Rules.
Keep in mind:
- The dropdown menu for setting conditions for a custom user property is not auto-filled — start typing a property name to find and select it.
- It is possible to create an audience using a property that has not yet arrived in Apphud - just type an expected value in the "...is equal to" field.
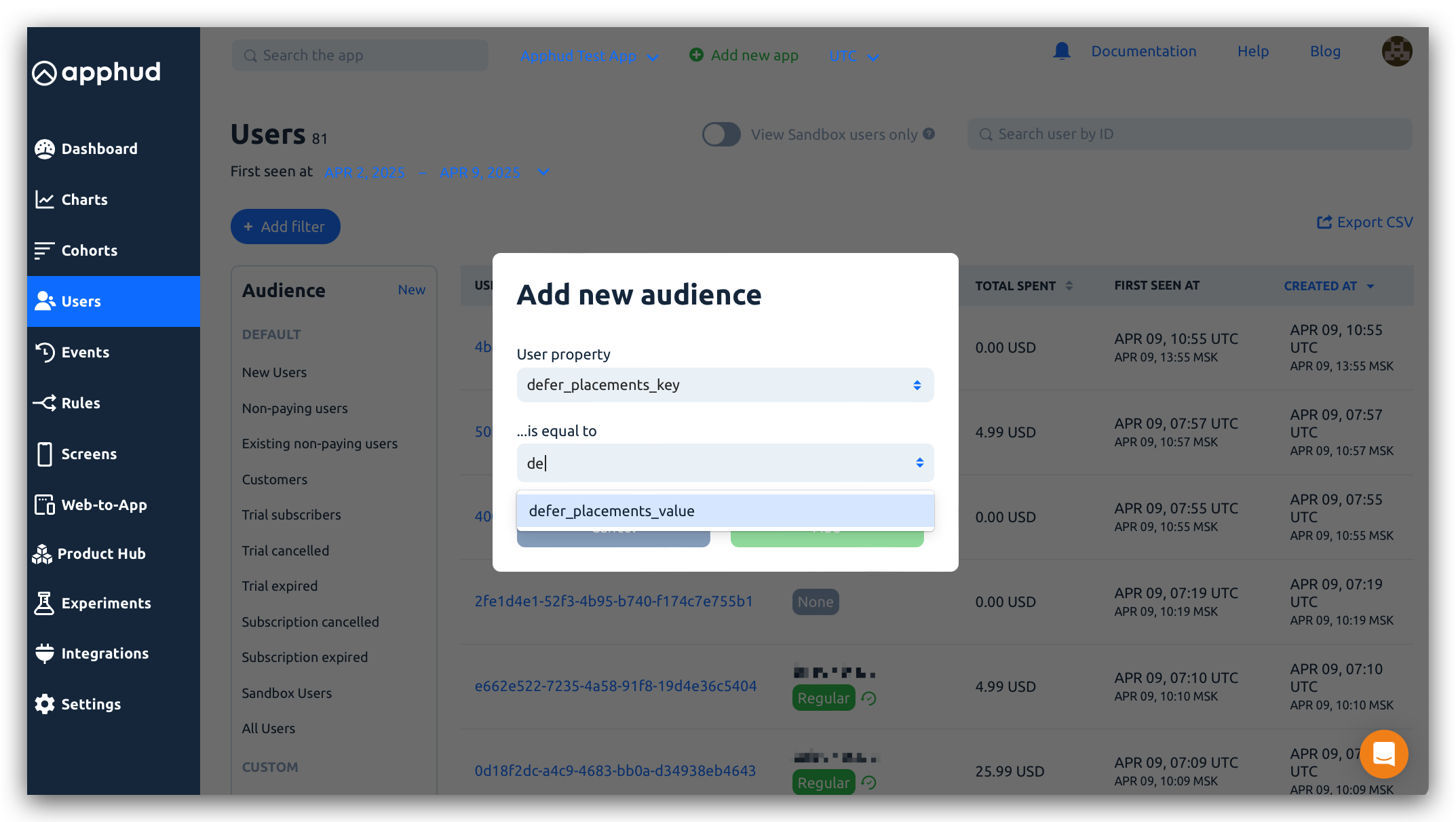
Placements, Experiments & Rules
Once audiences based on custom user properties are defined, it can power:
- Dynamic content delivery through Placements (e.g., showing a different paywall based on the audience)
- Targeted Experiments for A/B testing different flows
- Engagement with a certain user group defined by custom properties via Apphud Rules
By leveraging Custom User Properties across these tools, you can deliver more relevant experiences, analyze behavior in context, and boost monetization efficiency.
Updated about 1 month ago