Facebook Ads (Conversions API)
This guide describes how to add and configure Facebook Conversions API integration.
This is an improved version of Facebook Ads Integration
Current integration is using Conversions API and now supports App Events along with Web-to-App events and can be used for Aggregated Event Measurement. Supports both iOS and Android apps.
What is Conversions API?
The Facebook Conversions API is intended to establish a link between an advertiser's marketing information — including web events, app events, business messaging, and offline conversions — and Meta's systems. This connection is meant to enhance ad targeting, reduce costs per result, and improve measurement.
Apphud events are linked to a Dataset and are processed like events sent via the Meta Pixel, Facebook SDK for iOS or Android, mobile measurement partner SDK, offline event set, or .csv upload. This means that Apphud events may be used in measurement, reporting, or optimization in a similar way as other connection channels for both iOS and Android devices.
Support for Aggregated Event Measurement
Meta's Aggregated Event Measurement is a protocol that allows for measurement of web and app events from people using iOS 14.5 and later devices, including those who’ve opted out of tracking on Facebook or Instagram apps, while still respecting people’s privacy choices. Apphud Events sent to Facebook using Conversions API now support Aggregated Event Measurement.
How to add integration?
To get started with Conversions API integration you need to follow steps in the Events Manager:
-
Create Dataset ID if you haven't already. This can be done in Data Sources page in the Business Manager.
-
Link Dataset ID with your Facebook App ID. This can be done in the settings of your Data Source in Meta Events Manager.
-
Check app eligibility for Aggregated Event Measurement (iOS only). This can be done in the settings of your Data Source in Meta Events Manager. Your app must be eligible for App Events promotion.
-
Pass Device Attribution Data to Apphud. See below.
-
Generate Access Token. See below.
Pass Device Attribution Data to Apphud
Update to the latest Apphud SDKs
This is required step
If Apphud didn't receive device attribution from SDK, any subscription events from this device will not be forwarded to Facebook.
Passing device attribution data is required now:
// in future versions of Facebook SDK getting extinfo may change, update if necessary.
// if extinfo cannot be retrieved, pass at least anon id.
// call this after both Facebook and Apphud SDKs have been initialized
let extInfo = _AppEventsDeviceInfo.shared.encodedDeviceInfo
let anonId = AppEvents.shared.anonymousID
let data = ["extinfo": extInfo ?? ""]
Apphud.addAttribution(data: data, from: .facebook, identifer: anonId, callback: nil)
import com.facebook.appevents.AppEventsLogger
import com.facebook.internal.Utility
...
// in future versions of Facebook SDK getting extinfo may change, update if necessary.
// if extinfo cannot be retrieved, pass just anon id.
// call this after both Facebook and Apphud SDKs have been initialized
val json = JSONObject()
Utility.setAppEventExtendedDeviceInfoParameters(json, application)
val extInfo = json.get("extinfo")
val anonID = AppEventsLogger.getAnonymousAppDeviceGUID(application)
val map = mapOf("fb_anon_id" to anonID, "extinfo" to extInfo)
Apphud.addAttribution(ApphudAttributionProvider.facebook, map, anonID)
/*
Unfortunately, there isn't direct way to extract device attribution data from Dart code.
You will need to create custom channel and run native code on both iOS and Android platforms
https://docs.flutter.dev/platform-integration/platform-channels
Here is rough implementation of what you may need:
*/
//////// iOS:
// In your AppDelegate or a dedicated Swift file
import Flutter
import UIKit
import FBSDKCoreKit
// Ensure to implement FlutterPluginRegistrant in your AppDelegate if not already done.
private func setupFacebookChannel(with registrar: FlutterPluginRegistrar) {
let channel = FlutterMethodChannel(name: "com.yourcompany/facebook_sdk", binaryMessenger: registrar.messenger())
channel.setMethodCallHandler { (call, result) in
if call.method == "getFacebookData" {
let extInfo = _AppEventsDeviceInfo.shared.encodedDeviceInfo ?? ""
let anonId = AppEvents.shared.anonymousID ?? ""
result(["extinfo": extInfo, "anon_id": anonId])
} else {
result(FlutterMethodNotImplemented)
}
}
}
// Call setupFacebookChannel in your application didFinishLaunchingWithOptions
////// Android
// In your MainActivity or a dedicated Kotlin file
import io.flutter.plugin.common.MethodChannel
import io.flutter.plugin.common.PluginRegistry.Registrar
import com.facebook.appevents.AppEventsLogger
fun setupFacebookChannel(registrar: Registrar) {
val channel = MethodChannel(registrar.messenger(), "com.yourcompany/facebook_sdk")
channel.setMethodCallHandler { call, result ->
if (call.method == "getFacebookData") {
val json = JSONObject()
Utility.setAppEventExtendedDeviceInfoParameters(json, applicationContext)
val extInfo = json.get("extinfo").toString()
val anonID = AppEventsLogger.getAnonymousAppDeviceGUID(applicationContext)
result.success(mapOf("extinfo" to extInfo, "fb_anon_id" to anonID))
} else {
result.notImplemented()
}
}
}
// Call setupFacebookChannel in your MainActivity's onCreate method
/// Flutter (Dart)
import 'package:flutter/services.dart';
class FacebookSDK {
static const _channel = MethodChannel('com.yourcompany/facebook_sdk');
static Future<Map<String, dynamic>> getFacebookData() async {
final Map<String, dynamic> data = await _channel.invokeMethod('getFacebookData');
return data;
}
}
/*
Then, you can call FacebookSDK.getFacebookData() from your Flutter code
to get the extInfo and anonId.
Remember to handle the asynchronous nature of the platform channel call.
This is a simplified version. Depending on your application's architecture,
you might need to adjust the code for error handling, initialization, and other aspects.
Also, make sure your platform-specific code is up-to-date
with the latest SDK versions and best practices.
*/
Generate Access Token
To generate Access Token find Generate access token button in the settings of your Data Source in Meta Events Manager. Note that access token is displayed only once. You will not be able to view the token once the page is refreshed.
Keep in mind
You can re-generate access token and update the value in the integration settings in Apphud at any time.
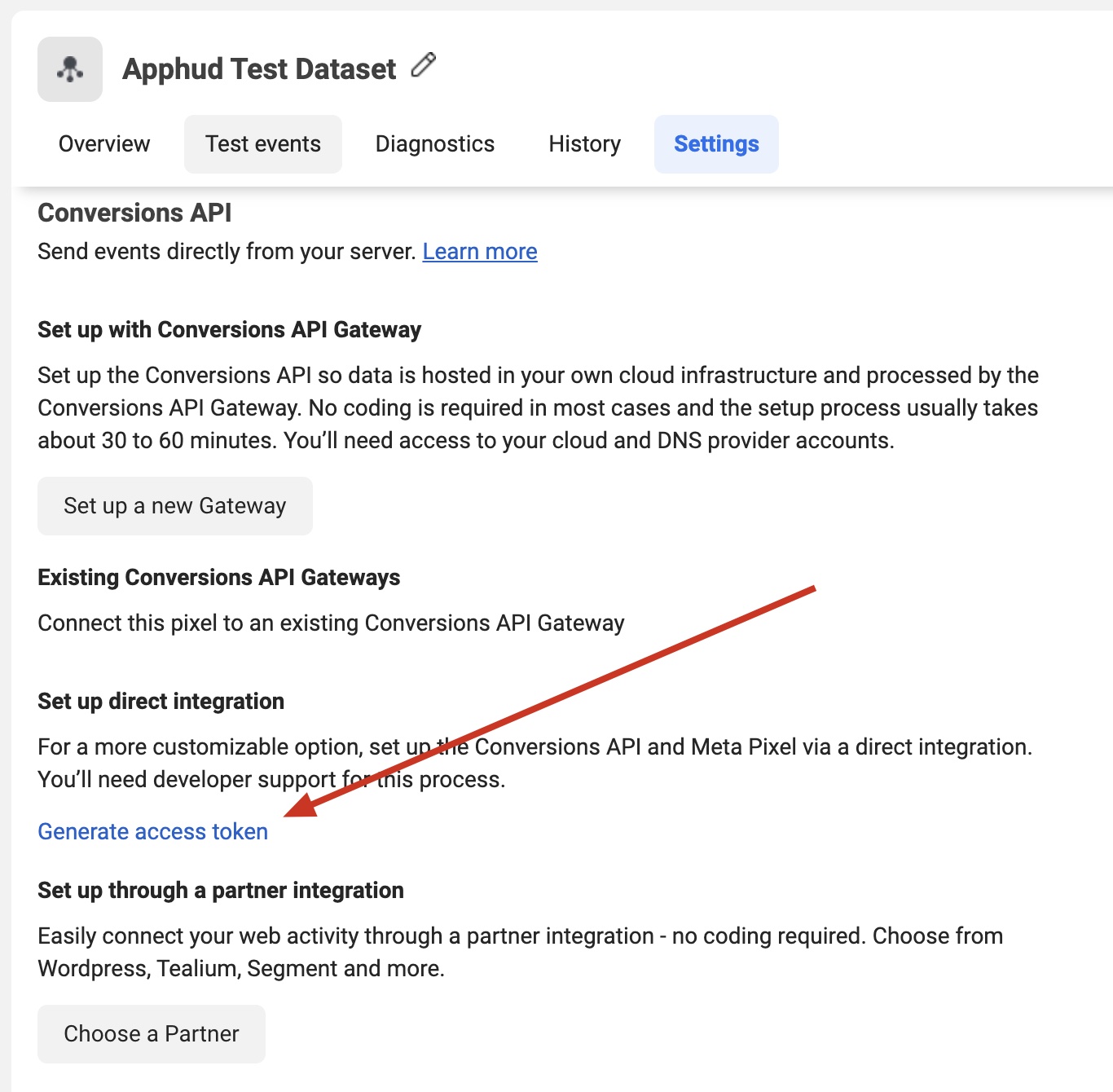
How to add integration?
Step 1
- Go to Apphud integrations page and select Facebook Ads (Conversions API) integration.
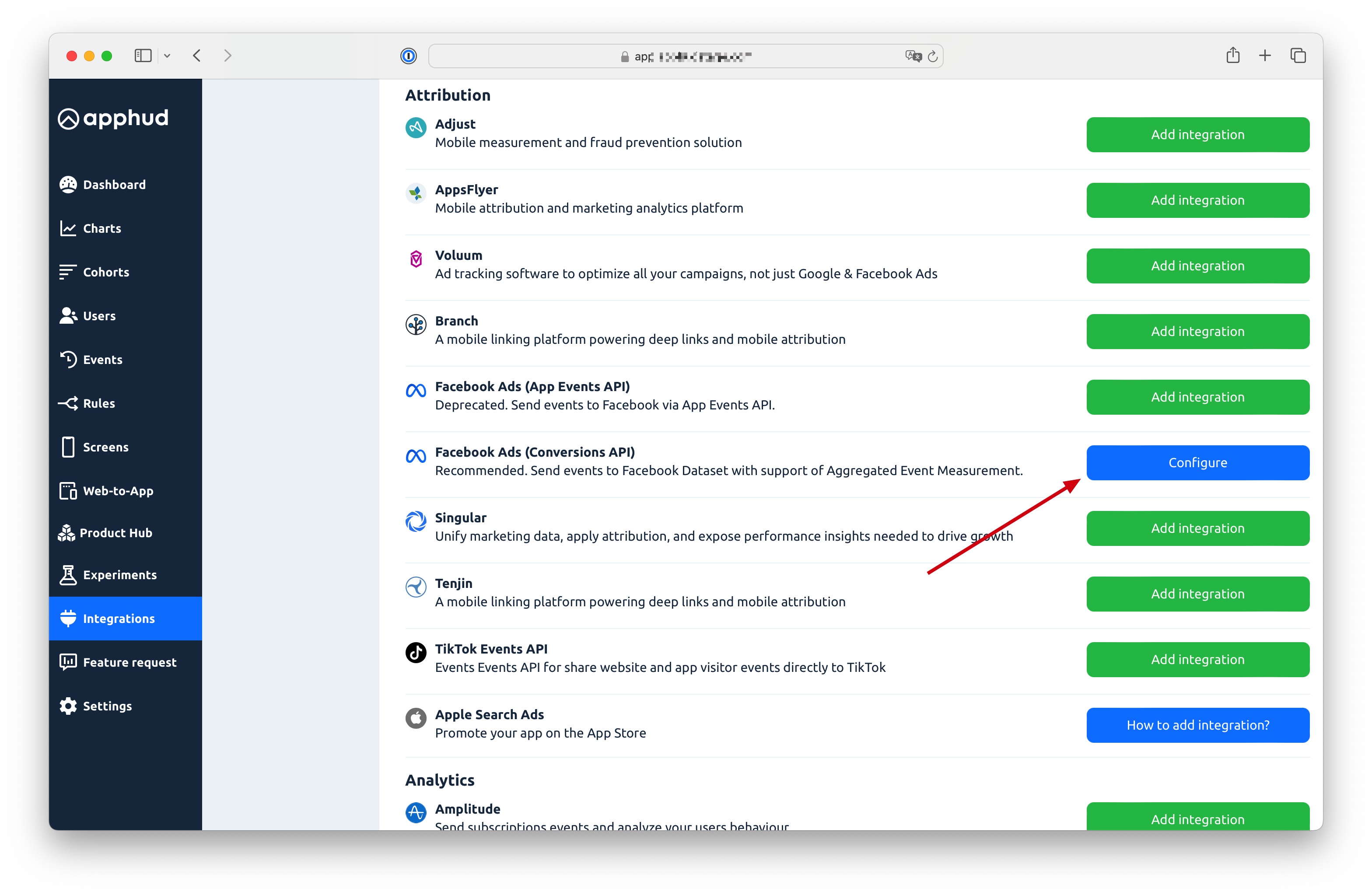
Step 2
- Enter your Dataset ID (you can get in the settings of your Data Source in Events Manager)
- Enter your Access Token (you can generate it in the settings of your Data Source in Events Manager)
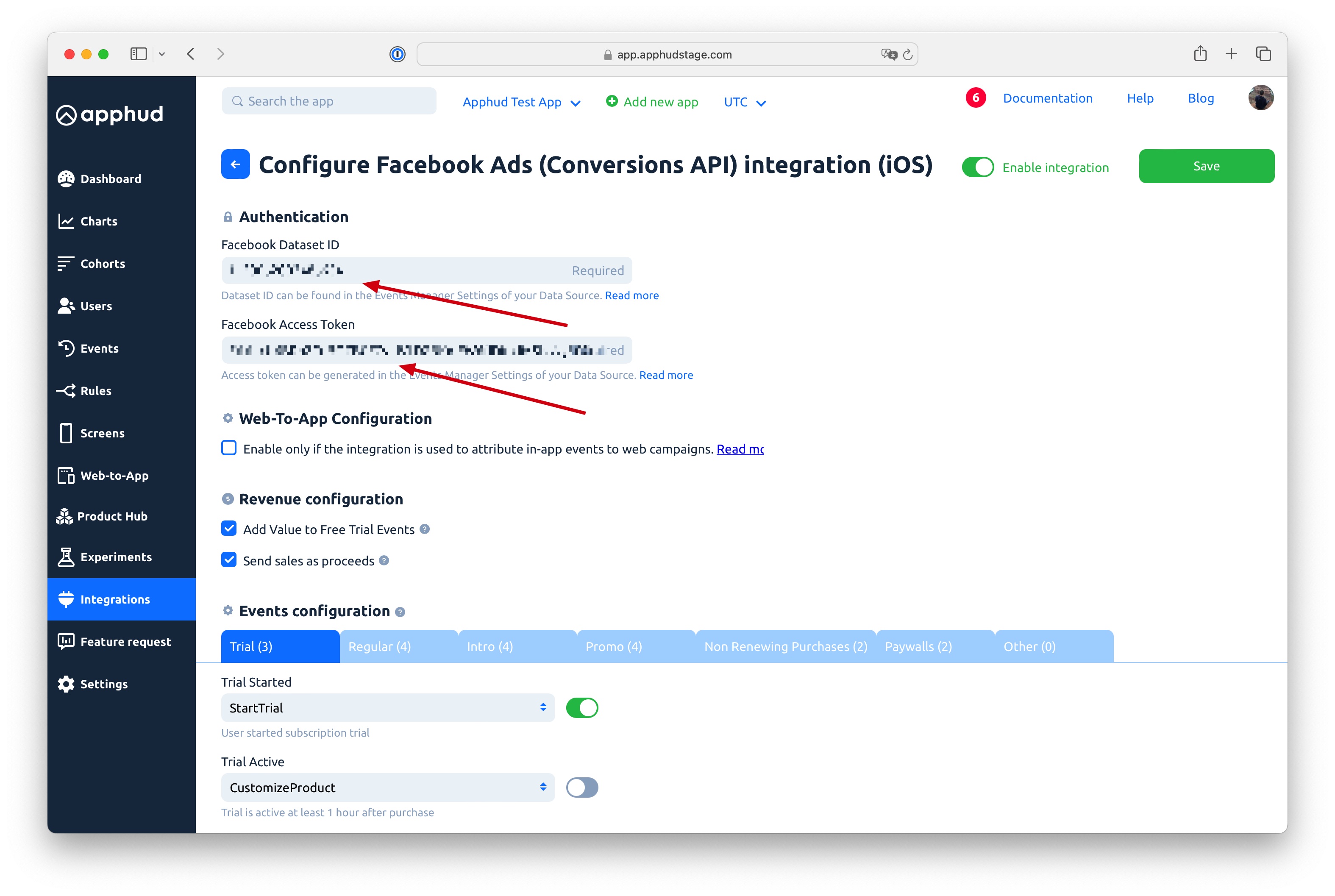
Step 3
- Configure events mapping. Apphud Events must be mapped to one of Facebook Standard Events. You can disable events that you don't need.
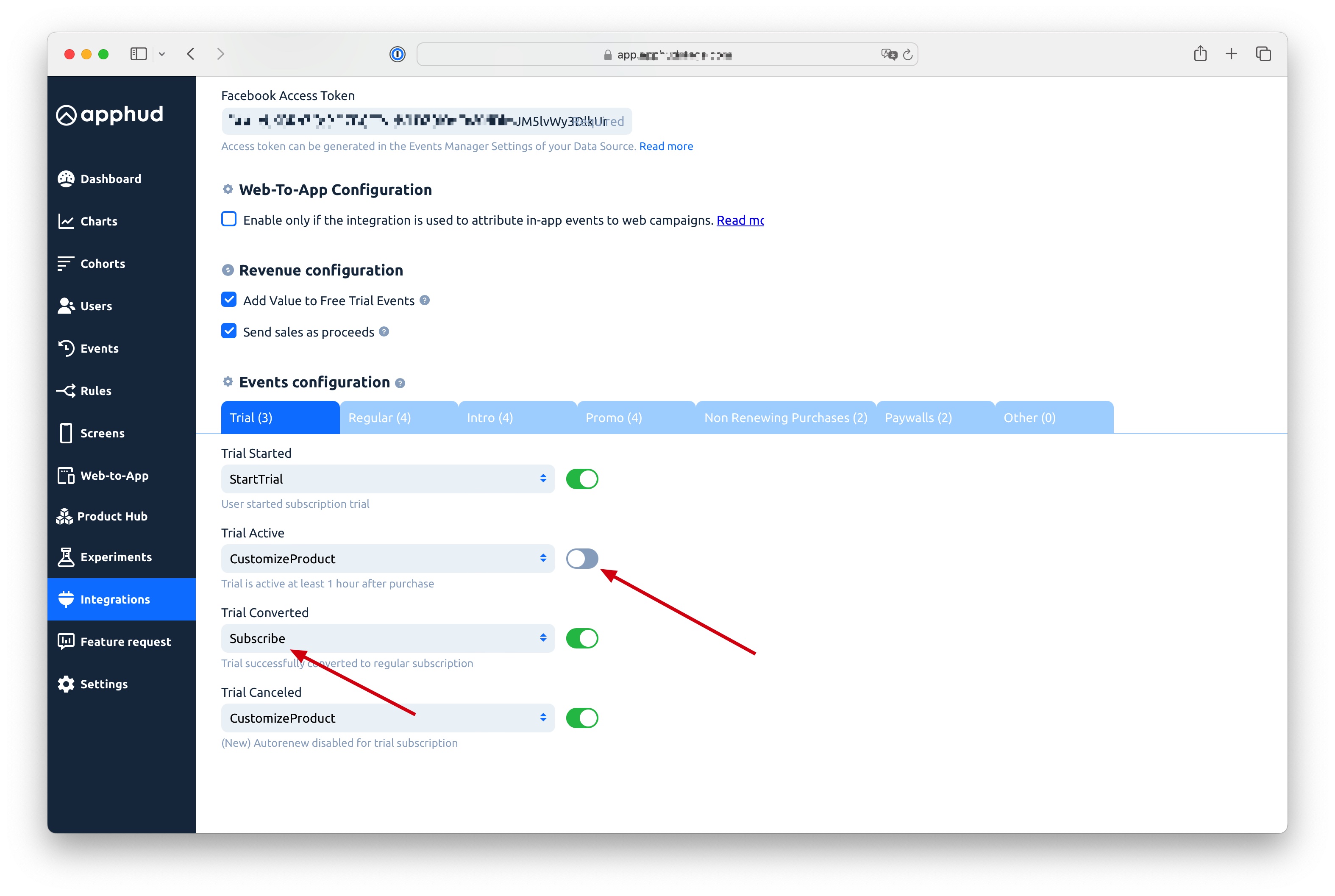
Step 4
- Enable Integration and Save.
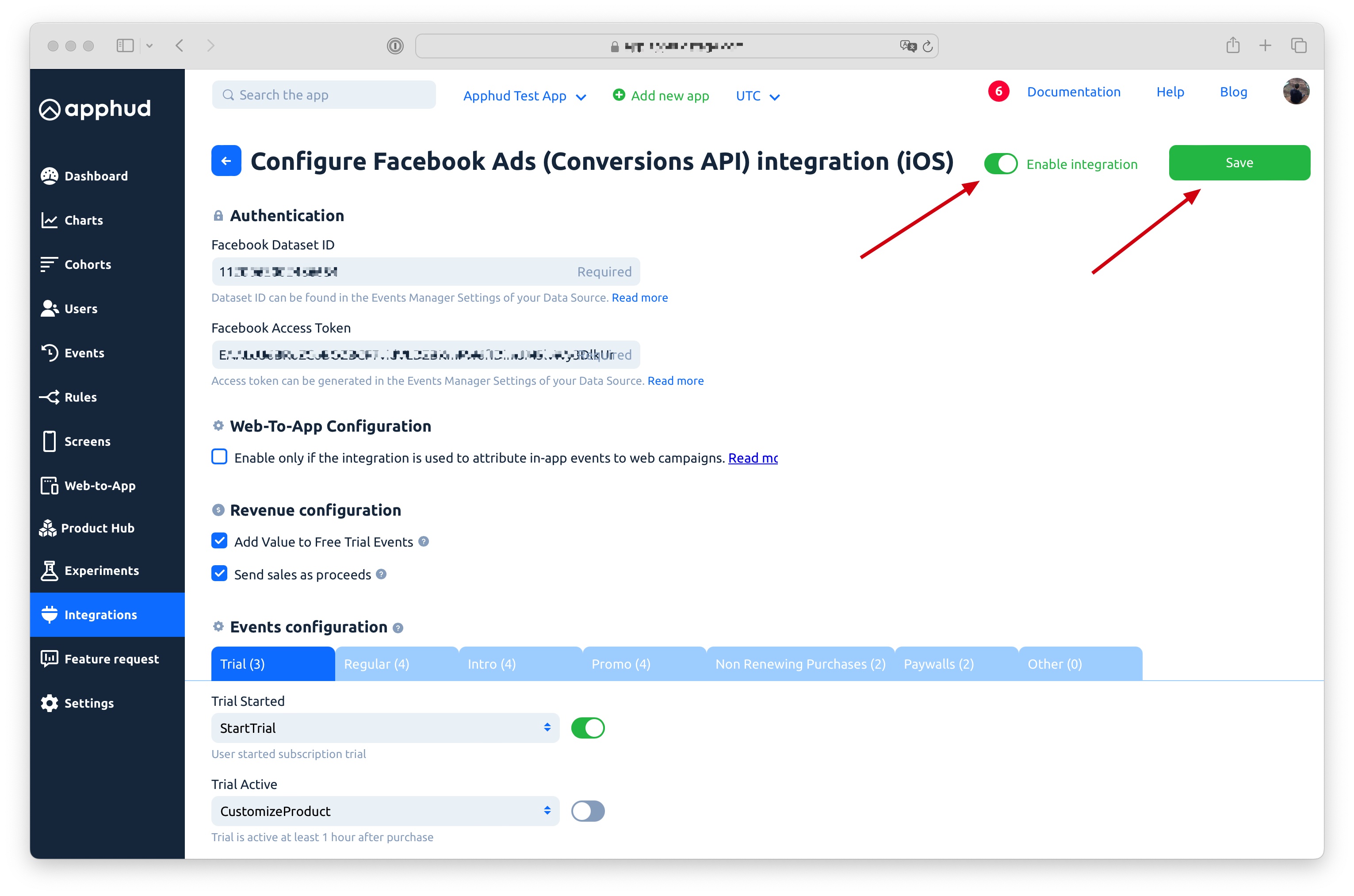
Validate Setup
- If everything works correctly, you should start seeing Apphud events sent by Conversions API in the Events Manager:
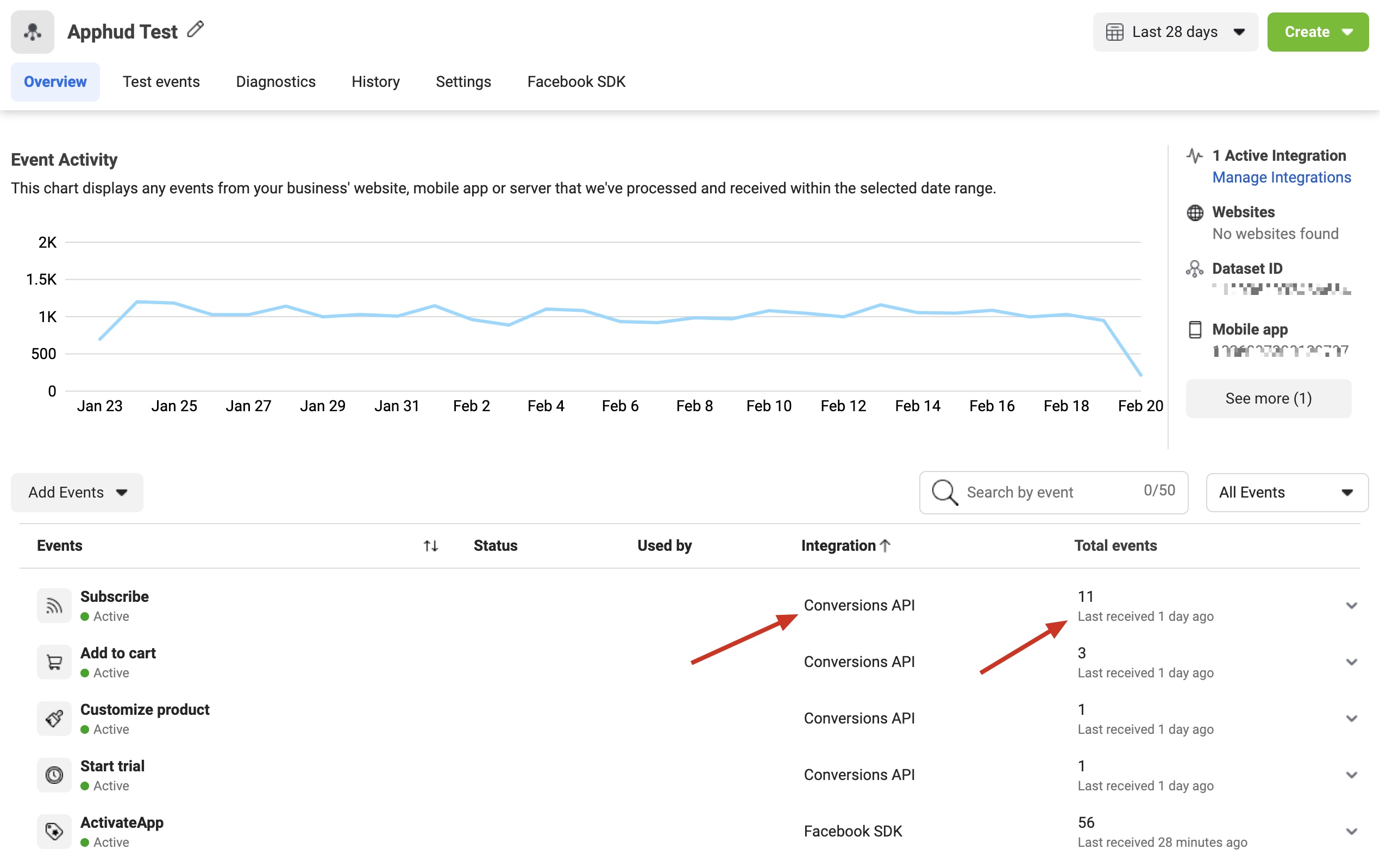
- Your App Event sent via Conversions API should be eligibile for Aggregated Event Measurement (iOS only). You can check this in the settings of your Data Source in Meta Events Manager.
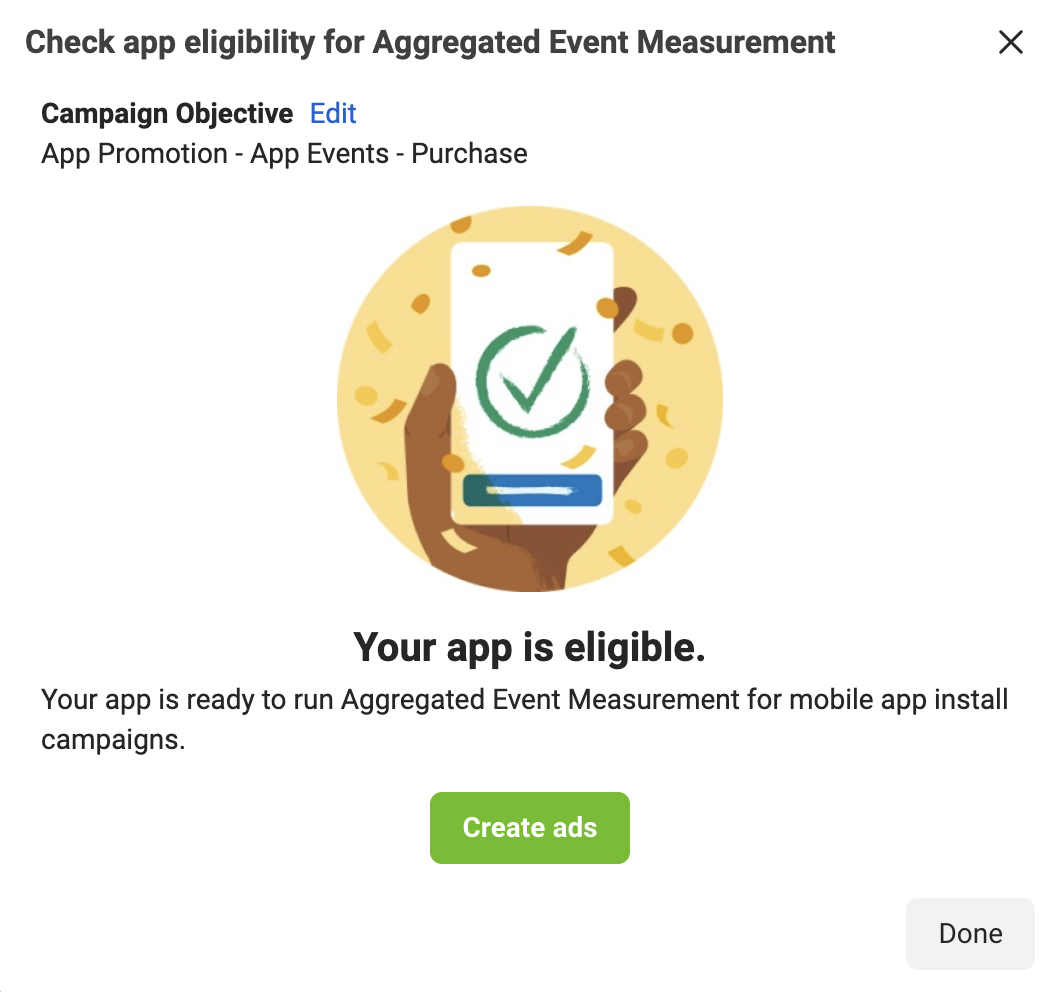
Using Meta's Aggregated Event Measurement Attribution
In the ad campaign set up, select Meta's attribution instead of SKAdNetwork:
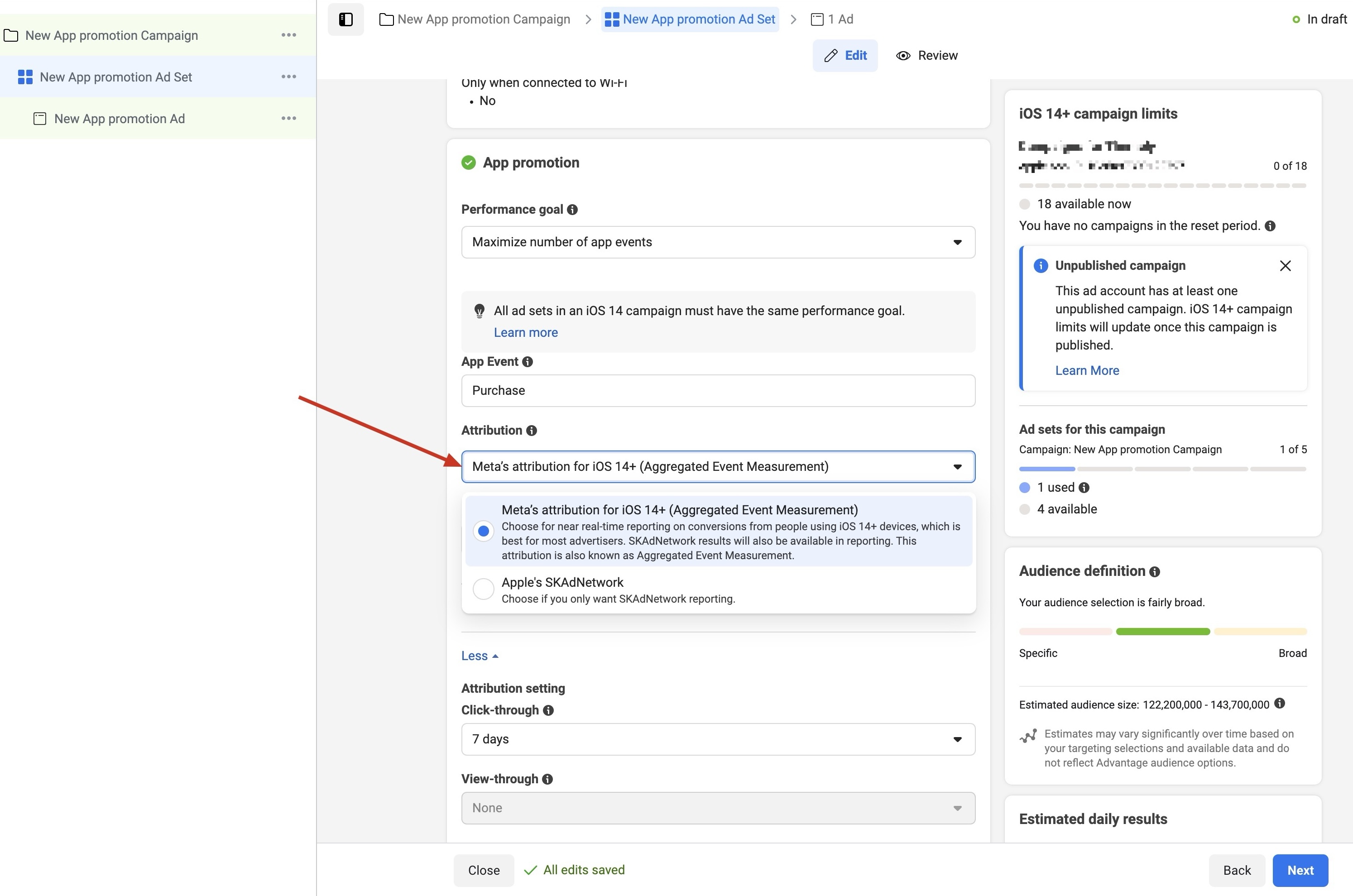
Using Conversions API for Web-to-App campaign optimization
Web-to-App integration
All the documentation below is related to Web-to-App campaigns
Conversions API integration can also be used to run Web-to-App (or Web2App) campaigns in Facebook with event optimization. Learn more.
Web-to-App campaign is a web campaign targeted to your app’s landing page which has a link to the App Store.
To use integration for Web-to-App campaigns, update it by checking the checkbox as in the screenshot below:
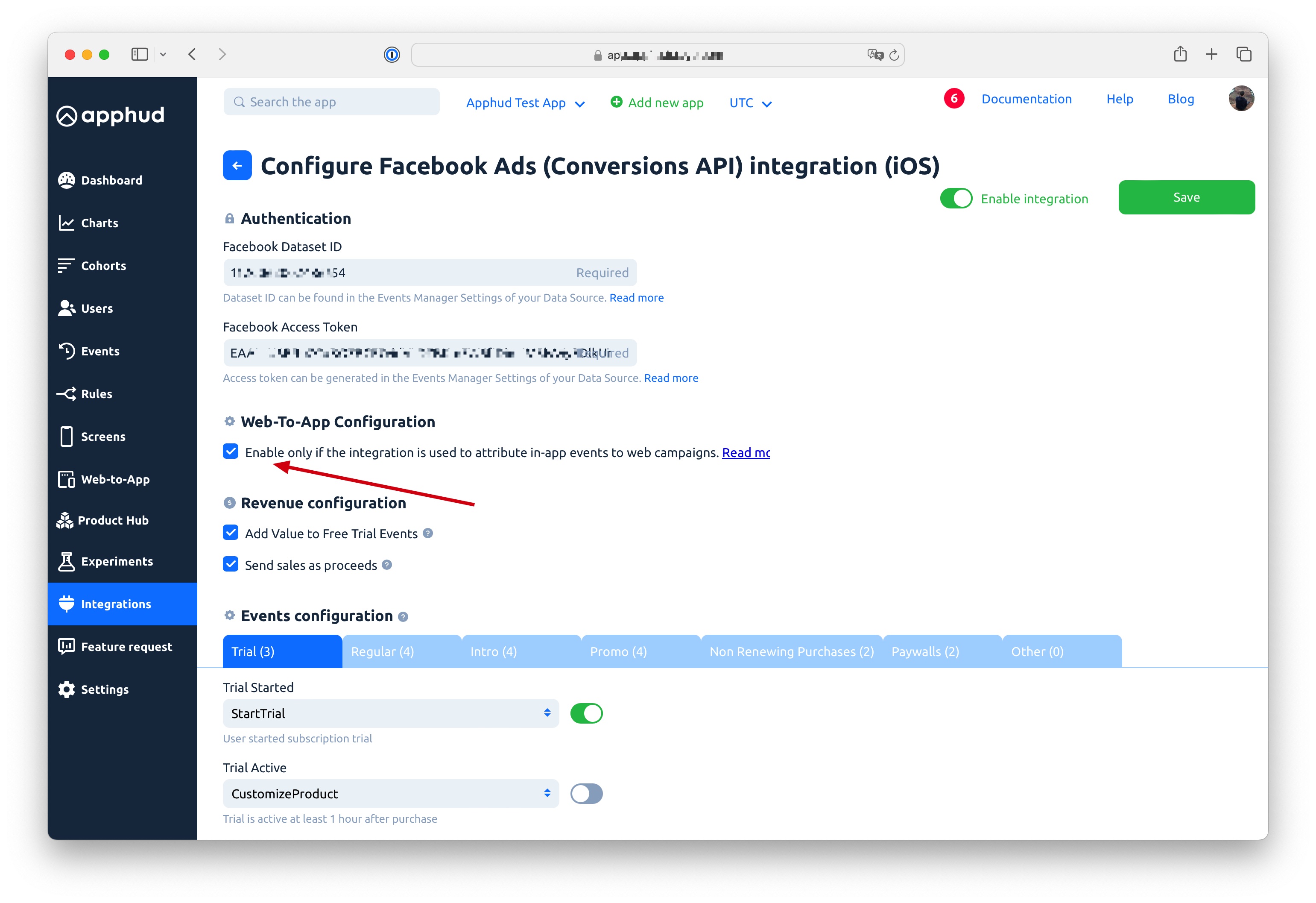
After saving you will see Apphud Pixel Script:
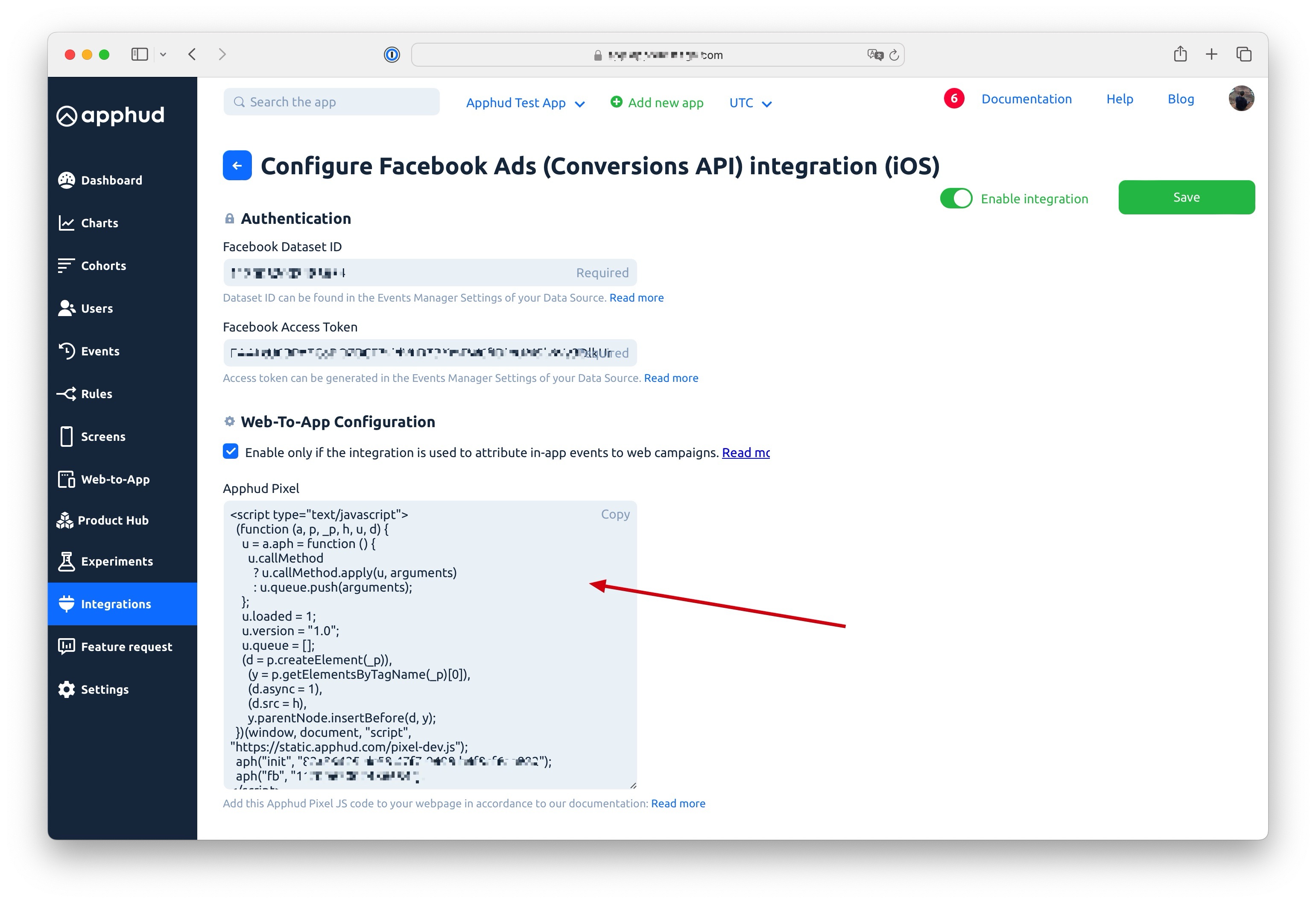
You will need to use this Script code only if you are using your own landing page for web campaigns. If you're planning to use our Landing Pages generated in the Landing Page Editor, you can skip a script installation step, since the script will be already installed.
Install Apphud Pixel to your Landing Page
Follow this guide to Install Apphud Pixel script on your own landing page.
For more information about Web-to-App campaigns visit this link.
Additional Web-to-App Parameters
You can add custom parameters, like email or date of birth, in order to increase match quality. Here is a list of acceptable additional optional parameters that you can send from Apphud SDK via user properties method for your Web-to-App campaigns:
email | Email of the user | Apphud.setUserProperty(key: .email, value: "[email protected]", setOnce: true) |
gender | male or female . Note: valid only if first name or last name provided. | Apphud.setUserProperty(key: .gender, value: "male", setOnce: true) |
fb_login_id | The ID issued by Facebook when a person first logs into an instance of an app. This is also known as App-Scoped ID | Apphud.setUserProperty(key: .init("fb_login_id"), value: "someID") |
birthdate | Date of birth in YYYYMMDD format, example: 19970216 | Apphud.setUserProperty(key: .init("birthdate"), value: "19970216") |
first_name | First name of the user. Note: valid only if gender provided. | Apphud.setUserProperty(key: .init("first_name"), value: "Thomas") |
last_name | Last name of the user. Note: valid only if gender provided. | Apphud.setUserProperty(key: .init("last_name"), value: "Anderson" |
For more information about customer parameters visit this link.
Receive Web-to-App Attribution Data
In order to get attribution data from Facebook web campaigns, you will need add URL parameters to understand where visitors are coming from.
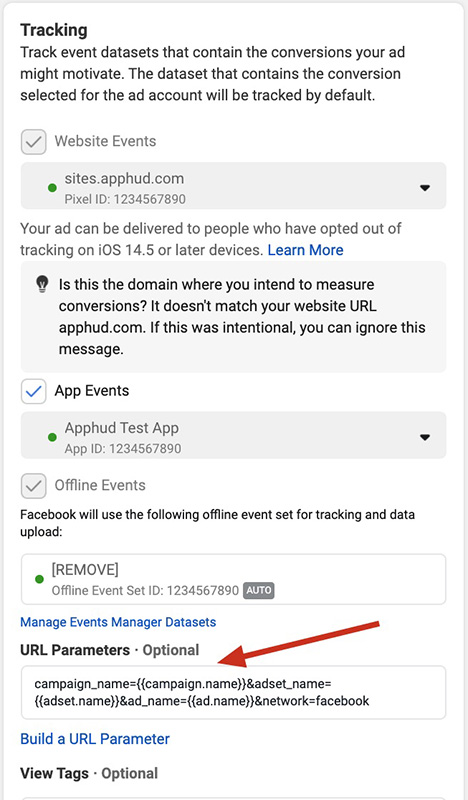
Paste these parameters to URL parameters field in Tracking section of your ad set up page.
campaign_name={{campaign.name}}&adset_name={{adset.name}}&ad_name={{ad.name}}&network=facebook
More information about URL parameters for attribution can be found here and here.
Analyze Web-to-App campaigns in Apphud
Since integration is a part of Web-to-App solution, analytics is described in this guide.
Troubleshooting
Please double check the following list in case you experience issues with integration. Make sure the following is correct:
- Your landing page should contain correct script code. Double check this guide.
- Events should be correctly mapped with Apphud events in integration settings page. For example,
Subscription Started
event should be mapped either withPurchase
orSubscribe
event. It's up to developer to choose desired mapping. - Double check Dataset ID is valid.
- Invalid Subscribe Value Parameter. One or more of your Subscribe events has invalid characters in the value field.
Try to disable refund events from integration setup. - Try to re-generate access token and update it in Apphud.
- Read Facebook's useful docs: https://www.facebook.com/business/help/721422165168355
- Still having troubles? Contact us.
- You may experience low install or purchase rate when running Web-to-App campaign with Purchase event optimization. Consider targeting for less priority events at the beginning, like
User Created
,Paywall Shown
orPaywall Checkout Initiated
. You can view and edit event mapping in Facebook Conversions API integration page.

By default, "User Created" event is mapped to Lead event. You can change this at any time.
- Event error:
Unsupported post request. Object with ID '1234567890' does not exist, cannot be loaded due to missing permissions, or does not support this operation.
Please make sure you entered correct Dataset ID, and access token was created by Facebook User that has correct permissions to view datasource settings in the Events Manager, this user must not be banned, deleted, etc. - Event error:
Error validating access token: The session has been invalidated because the user changed their password or Facebook has changed the session for security reasons.
Please re-generate your access token.
Updated 6 days ago